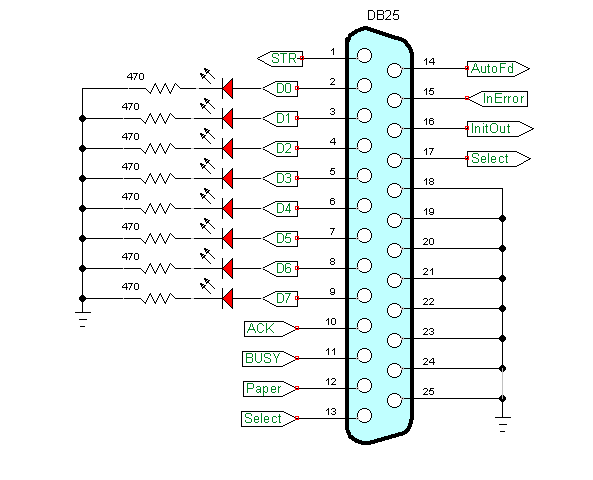
Reading TLC548 Serial ADC Through PC Printer Port Part 1
So far in this series we've learned bit operations including shift operations, turning LEDs on-off, detecting a switch connected to an input on the PC printer port. All of this now comes together as we use a TLC548 8-bit serial analog to digital converter to read an external voltage through the PC printer port.
The 8-bit result will be displayed on the 8 LEDs we connected to the data port (above) and printed out on the terminal.
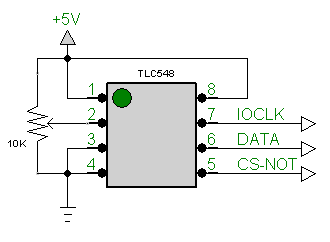
The TLC548 is an 8-pin DIP ADC is wired with a pot as shown above. Note the following connections:
p.setDataStrobe(0) # Pin 1 output to CLK pin 7 TLC548
p.getInError() # Pin 15 input to DATA OUT TLC548 pin 6.
p.setInitOut(1) # Pin 16 output to CS-NOT pin 5 TLC548
There are three connections to the PC printer port. Pin 1 Strobe is an output that acts as a serial clock. Pin 15 Error or fault is an input whose state is read to determine its state in relation to the serial clock pulse.
The data byte transmitted from the ADC is least significant bit first thus need to be shifted left during the read cycle. Pin 16 Init is an output the enables the TLC548 for a serial data read.
def readADC(): temp = 0 p.setInitOut(0) # enable CS # time.sleep(.01) for i in range(0,7): # loop here 7 times p.setDataStrobe(1) # CLK HIGH temp = temp + p.getInError() # get bit temp = temp << 1 # shift 1 bit left p.setDataStrobe(0) # CLK LOW p.setDataStrobe(1) # CLK HIGH temp = temp + p.getInError() # get bit 8 p.setDataStrobe(0) # CLK LOW p.setInitOut(1) # CS HIGH return temp
The routine readADC() is simply a "for" that enables the chip, toggles the CLK bit (Strobe) then reads each bit shift adding a 1 or 0 to temp shift left to reconstruct the ADC data. The data byte is returned to a variable; ADCval = readADC().
# When Sw1 pressed program exits. while Sw1(): val = readADC() p.setData(val) # send to 8-bit port LEDs time.sleep(.1) print "Reading =", convBinary(val), " ", hex(val)
The short program above receive the data byte from readADC() and prints the value in binary and hex.
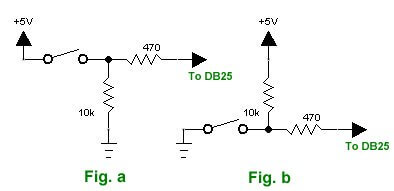
The switch in Fig. b is connected to Db25 pin 10 and when pressed exits the program.
The TLC548 is available for under $4 from Newark Electronics.
Other projects on this site using the TLC548:
- Connecting the TLC548 ADC to the PICAXE
- Using a Microchip PIC with TLC548 Serial ADC
- TLC548 Serial ADC Spec. Sheet
Next we'll alter the pin connections to the TLC548 to use data port pins D0 and D1 to free up pins to connect a serial LCD display in the following project. This also demonstrated manipulating individual bits on the 8-bit data port.
See Reading an Analog Voltage Through the PC Printer Port Part 2
The program is written in Python and runs under Linux. To use this one must setup a module pyparallel. How to set this up is on my webpage Programming the PC Printer Port in Python
#!/usr/bin/env python # File pporttlc548.py # http://www.bristolwatch.com/pport/index.htm # By Lewis Loflin - lewis@bvu.net # Read serial ADC TLC548 and send value # to 8 LEDs on pins 2-9 on Db25. # As one adjusts pot binary values displayed on LEDs # and terminal window. # Program halts when Sw1 is pressed. # Sw1 is connected from ground to Db25 pin 10 and pulled high # by a 10k resistor. import parallel import time p = parallel.Parallel() ''' ; Pins on the TLC548 8-bit ADC: ; 1 -> Ref + -> connect to Vcc ; 2 -> analog in ; 3 -> Ref - -> connect to GND ; 4 -> GND ; 5 -> CS-NOT -> chip select active LOW ; 6 -> DATA OUT ; 7 -> CLK ; 8 -> Vcc ''' # init i/o pins p.setDataStrobe(0) # Pin 1 output to CLK pin 7 TLC548 p.setAutoFeed(0) # Pin 14 output NC p.getInError() # Pin 15 input to DATA OUT TLC548 p.setInitOut(1) # Pin 16 output to CS-NOT pin 5 TLC548 p.setSelect(0) # Pin 17 output NC # data LSB first # enable TLC548 CS-NOT LOW # set CLK HIGH # read data bit shift left # set CLK LOW def readADC(): temp = 0 p.setInitOut(0) # enable CS # time.sleep(.01) for i in range(0,7): # loop here 7 times p.setDataStrobe(1) # CLK HIGH temp = temp + p.getInError() # get bit temp = temp << 1 # shift 1 bit left p.setDataStrobe(0) # CLK LOW p.setDataStrobe(1) # CLK HIGH temp = temp + p.getInError() # get bit 8 p.setDataStrobe(0) # CLK LOW p.setInitOut(1) # CS HIGH return temp # convert a 8-bit number (integer) to a binary. # Returns string. # unlike python bin() this doesn't drop leading zeros def convBinary(value): binaryValue = 'b' for x in range(0, 8): temp = value & 0x80 if temp == 0x80: binaryValue += '1' else: binaryValue += '0' value = value & 1 return binaryValue def Sw1(): return p.getInAcknowledge() # check pin 10 Db25 when pressed returns 0 # Sw1 is connected from ground to Db25 pin 10 and pulled high # by a 10k resistor. # When Sw1 pressed program exits. while Sw1(): val = readADC() p.setData(val) # send to 8-bit port LEDs time.sleep(.1) print "Reading =", convBinary(val), " ", hex(val) p.setData(0) # LEDs off print print "Exit now." exit
Download pport-1.0.iso from Sourceforge.com then burn to DVD (file size 920 meg.), insert into DVD drive and reboot. Make sure PC is set to boot from DVD ROM.
This is pre-configured by myself to use Python to control the printer port. Python can be run from IDLE or Geany.
All of my PPORT electronics projects will work without installation to a PC.
Programs can be saved to thumb drive in LIVE mode.
Projects
Below are listed a series of projects using pyparallel and electronics. Starting with routines I wrote to aid students I'd advise walking through this in sequence. Have fun and send comments and/or corrections to lewis@bvu.net.
- Introduction to Python Bitwise Operations
- Python Bitwise Operations by Example
- Using the PC Printer Port series:
- Programming the PC Printer Port in Python
- Additional Commands for Py-Parallel
- Controlling Data Bits on the PC Parallel Port
- Connecting Switches to the PC Printer Port with Python
- Reading an Analog Voltage Through the PC Printer Port Part 1
- Reading an Analog Voltage Through the PC Printer Port Part 2
- Controlling a Serial LCD Display on a PC Printer Port with Python
- Serial ADC and LCD Display with PC Printer Port with Python
- Controlling MAX7219 LED Display with PC Printer Port with Python
- MAX7219 8-Digit LED Display and Serial ADC in Python
- Project pages:
- Part 1: Read Arduino with PC Printer Port
- Part 2: Better way to Read Arduino Through the PC Printer Port
- Part 3: Read-Write an Arduino Through a PC Printer Port
- Part 4: Control LCD Display and Arduino from the PC Printer Port
- Arduino sketches needed by programs:
- pportArduino1.ino read only after reset.
- pportArduino2.ino reset once and multiple reads.
- pportArduino3.ino reset once read write Arduino infinite times - multiple commands.
- Related Raspberry Pi projects:
- Connect Serial LCD to Raspberry Pi
- Serial Read from Arduino to Raspberry Pi
- Arduino Raspberry Pi Interface with LCD Display
Linux Videos
- Live Linux Distro for Using Printer Port with Electronics
- Using the powerful Rox-Filer system in Linux
- Use FEH under Linux for a Wallpaper Setter
- How to create Symbolic links in Linux
- Printer Port Interfacing Videos:
- Connect Electronics to PC printer Port with Python
- Setup PC Printer Port with Python-Linux
- Use PC Printer Port to Read Analog Voltage
- Read-Write Arduino ADC PWN with Printer Port
- Printer Port to Serial LCD Display
- Connect Arduino to PC Printer Port for advanced control
Printer Port in C
- Exploring Digital Computer Electronics
- Hardware Review Connecting PC Parallel Ports
- Operation TB6600 Stepper Controller with PC Parallel Port
- Build or Buy Parallel Port Breakout Board?
- Build Serial HD44780 LCD Display Connect to Parallel Port
- Motherboards
- Presario 1999 CM1001 Gaming Computer Salvage
- Live Test 2002 VIA EPIA-800 Mini ITX Motherboard
- Salvage, Test 2012 AAEON EMB-B75A Industrial Motherboard
- Web Master
- Gen. Electronics
- YouTube Channel
- Arduino Projects
- Raspberry Pi & Linux
- PIC18F2550 in C
- PIC16F628A Assembly
- PICAXE Projects
Web site Copyright Lewis Loflin, All rights reserved.
If using this material on another site, please provide a link back to my site.